Master Basic Mathematics in Python
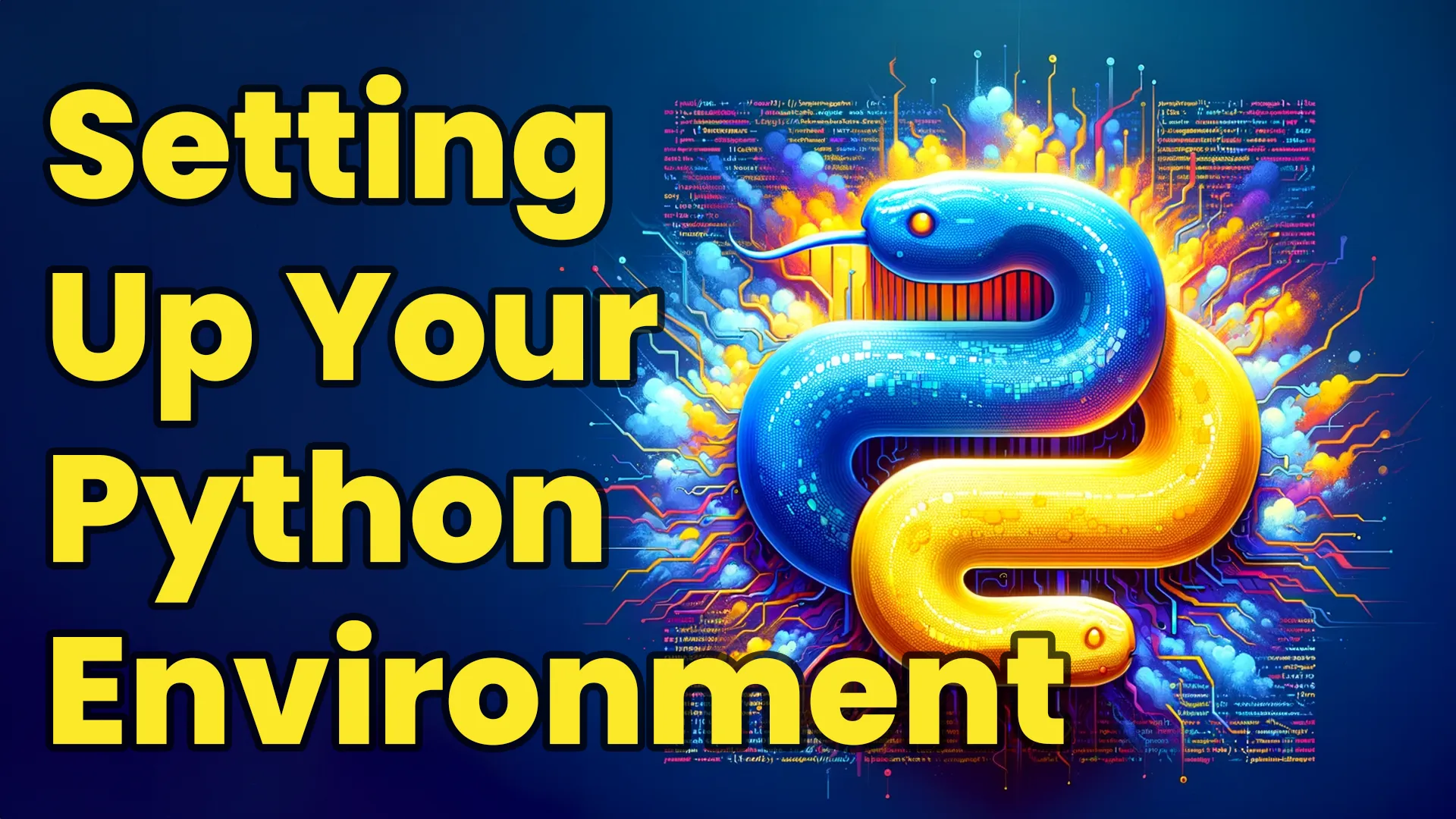
Overview
The learning objectives for this python lesson are as followings:
- basic mathematical operations
- order of operations
- integer versus float
- variable naming rules
- print function
- comments
Operations
How many mathematical operations can you think of? Four? Five? Well there are more than that. In this lesson we are going to be learning about the seven most common ones.
operation | symbol | example | result |
---|---|---|---|
exponent | ** | 2 ** 3 | 8 |
modulus/remainder | % | 5 / 2 | 1 |
multiplication | * | 2 * 3 | 6 |
division | / | 4 / 2 | 2.0 |
integer division | // | 4 / 2 | 2 |
addition | + | 1 + 2 | 3 |
subtraction | - | 2 - 1 | 1 |
Now in order to get some output that the use can actally see, we are going to take a look at a function in Python called print. We will take a deeper look what we can do with print later, but for now all we need to know is that we can use the print function to show a result to the user.
Let’s take a look at how it works.
2 + 2
Run the code and notice that nothing happens. Now enter the following code and press the run button.
print(2 + 2)
You should get the optput of 4 in your terminal this time. What is happening is Python will do the math foro us and then the print function will print the result out to us so long as we have the math parts in the between the parentheses.
—< EXAMPLES HERE >—
print(2 + 2) # 4
print{2 - 1 * 3) # -1
Order of Operations
PENDAS is what the kidos are calling it these days. This is the order of operations that you need to follow when doing math. Parentheses, Exponents, Multiplation, Division, Addition and Subtraction. You may have noticed that there are a few operations that we have learned about that are missing. Below is a chart with each level of operations. Operations on the same level are done at the same priority, but remember to do them from left to right.
Letter | Operation |
---|---|
() | parentheses |
** | exponents |
/ * // % | division, multiplication, integer division, modulus |
+ - | addition, subtraction |
—< EXAMPLES HERE >—
Integers & Floats
We have looked at two types of numbers so far in this lesson. One with a decimal, and one with out. We have seen that we can use them together in mathimatical operations, but they are a little different.
type | meaning |
---|---|
integer | Any whole number. Positive or negative. |
float | Any number with a decimal in it. Positive or negative. |
—< EXAMPLES HERE >—
Three Rules For Naming Variables
While you can name a variable pretty much anything, there are three basic rules to follow.
- you can not have any spaces in the name
- it can not begin with a number
- it can only contain letters, numbers, and the underscore character _
Good | Bad | Reason |
---|---|---|
num2 | 2num | can not start with a number |
two_nums | two-nums | can not have a - in it |
two_nums | two nums | can not have a space in it |
BIG_NUMBERS | BIG*NUMBERS | can not have a * in it |
When picking a name for a variable, keep in mind what it represents. The name of the variable should describe what data is stored in it.
Tags:
#float
#int
#math
#operators
#order of operations
#python